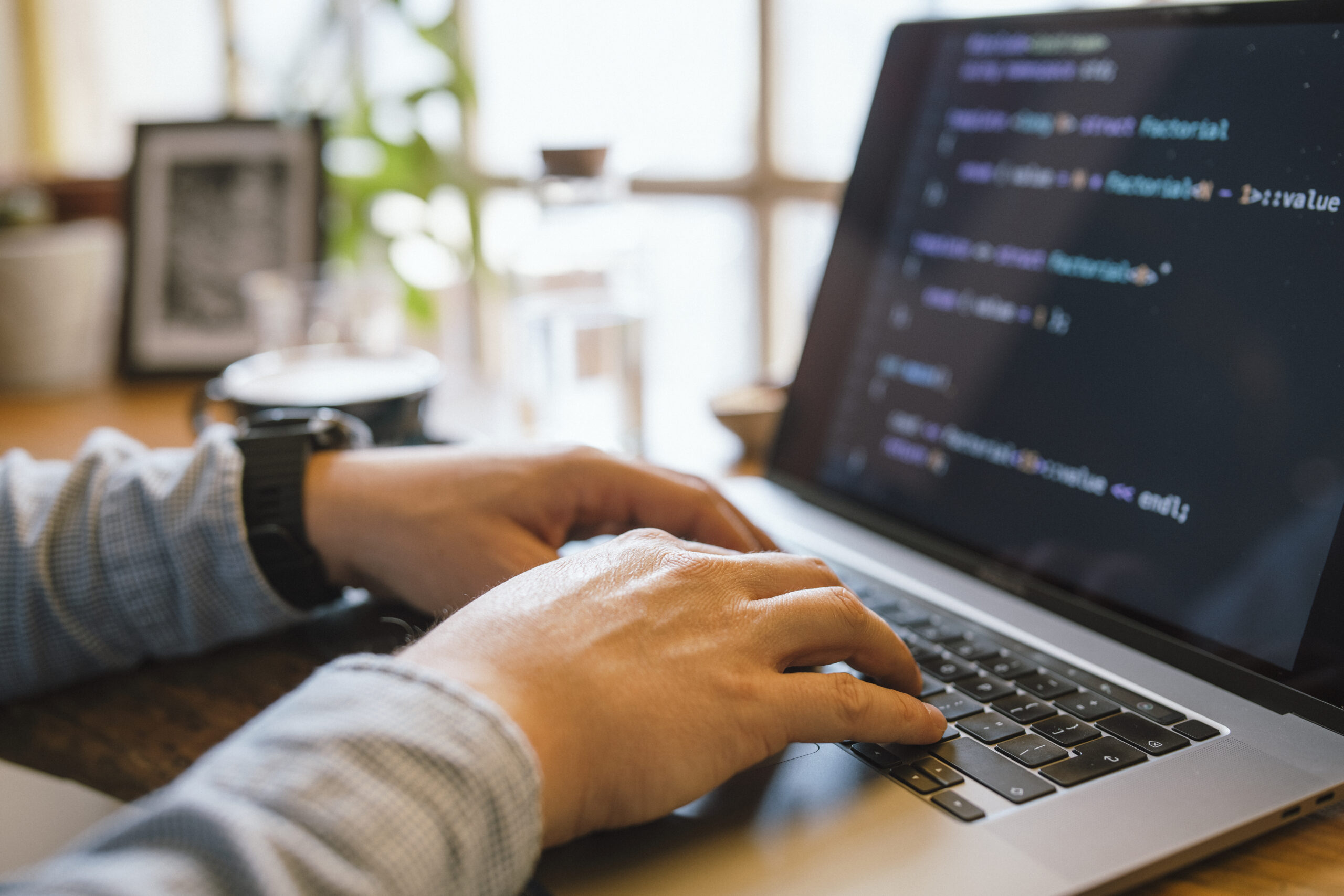
Debugging is Among the most vital — nonetheless frequently missed — abilities within a developer’s toolkit. It is not almost repairing damaged code; it’s about knowledge how and why matters go Completely wrong, and learning to think methodically to solve problems efficiently. Regardless of whether you're a beginner or perhaps a seasoned developer, sharpening your debugging abilities can conserve hours of frustration and dramatically improve your efficiency. Here i will discuss quite a few procedures to help builders amount up their debugging activity by me, Gustavo Woltmann.
Master Your Tools
One of the fastest means builders can elevate their debugging competencies is by mastering the applications they use everyday. Though crafting code is a person Component of growth, figuring out tips on how to communicate with it successfully during execution is Similarly crucial. Contemporary development environments occur Geared up with strong debugging capabilities — but many builders only scratch the floor of what these equipment can do.
Consider, by way of example, an Integrated Improvement Environment (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These equipment allow you to established breakpoints, inspect the value of variables at runtime, step as a result of code line by line, and in many cases modify code within the fly. When used accurately, they Allow you to notice just how your code behaves during execution, that's invaluable for monitoring down elusive bugs.
Browser developer resources, for instance Chrome DevTools, are indispensable for front-conclusion developers. They help you inspect the DOM, keep an eye on network requests, watch genuine-time general performance metrics, and debug JavaScript inside the browser. Mastering the console, resources, and network tabs can switch disheartening UI concerns into workable responsibilities.
For backend or method-stage builders, resources like GDB (GNU Debugger), Valgrind, or LLDB present deep control above functioning processes and memory management. Discovering these resources could have a steeper Discovering curve but pays off when debugging overall performance difficulties, memory leaks, or segmentation faults.
Further than your IDE or debugger, turn out to be relaxed with Variation Command systems like Git to grasp code heritage, obtain the exact moment bugs had been launched, and isolate problematic alterations.
In the long run, mastering your instruments usually means going beyond default settings and shortcuts — it’s about building an intimate understanding of your progress surroundings making sure that when challenges arise, you’re not lost in the dark. The better you realize your tools, the more time you can expend resolving the particular challenge as an alternative to fumbling by way of the method.
Reproduce the trouble
Just about the most vital — and often overlooked — ways in helpful debugging is reproducing the situation. Ahead of jumping into the code or making guesses, builders will need to make a steady atmosphere or state of affairs wherever the bug reliably appears. With out reproducibility, correcting a bug gets a recreation of chance, normally resulting in wasted time and fragile code variations.
Step one in reproducing a problem is accumulating as much context as possible. Check with queries like: What steps resulted in the issue? Which natural environment was it in — advancement, staging, or output? Are there any logs, screenshots, or mistake messages? The more element you may have, the less difficult it becomes to isolate the exact conditions underneath which the bug occurs.
As soon as you’ve collected ample info, endeavor to recreate the issue in your neighborhood atmosphere. This might mean inputting precisely the same data, simulating related person interactions, or mimicking program states. If The difficulty appears intermittently, look at creating automatic tests that replicate the edge scenarios or state transitions included. These checks not simply help expose the challenge but will also stop regressions Sooner or later.
Sometimes, the issue can be environment-certain — it would transpire only on certain working programs, browsers, or less than particular configurations. Making use of equipment like Digital equipment, containerization (e.g., Docker), or cross-browser tests platforms can be instrumental in replicating this sort of bugs.
Reproducing the challenge isn’t merely a move — it’s a mindset. It demands persistence, observation, plus a methodical tactic. But as you can regularly recreate the bug, you are presently halfway to repairing it. Using a reproducible situation, You need to use your debugging instruments additional proficiently, take a look at opportunity fixes properly, and communicate much more clearly together with your team or users. It turns an summary criticism right into a concrete problem — and that’s in which developers prosper.
Browse and Have an understanding of the Mistake Messages
Mistake messages are frequently the most precious clues a developer has when one thing goes Mistaken. As opposed to viewing them as frustrating interruptions, builders really should understand to deal with error messages as immediate communications through the program. They frequently tell you what precisely took place, in which it happened, and in some cases even why it took place — if you know how to interpret them.
Start by looking at the concept cautiously As well as in total. Numerous builders, particularly when under time force, glance at the main line and quickly begin creating assumptions. But further inside the mistake stack or logs may possibly lie the true root bring about. Don’t just copy and paste mistake messages into engines like google — read and fully grasp them initial.
Crack the error down into areas. Is it a syntax error, a runtime exception, or a logic error? Will it position to a specific file and line variety? What module or function induced it? These thoughts can guidebook your investigation and issue you toward the dependable code.
It’s also helpful to grasp the terminology in the programming language or framework you’re working with. Error messages in languages like Python, JavaScript, or Java usually comply with predictable styles, and Understanding to acknowledge these can drastically quicken your debugging course of action.
Some errors are obscure or generic, As well as in Those people instances, it’s critical to look at the context in which the error transpired. Test related log entries, input values, and recent improvements in the codebase.
Don’t neglect compiler or linter warnings both. These typically precede larger sized problems and provide hints about likely bugs.
In the long run, mistake messages are not your enemies—they’re your guides. Studying to interpret them appropriately turns chaos into clarity, supporting you pinpoint difficulties quicker, minimize debugging time, and become a far more successful and self-confident developer.
Use Logging Correctly
Logging is One of the more powerful tools inside a developer’s debugging toolkit. When utilized efficiently, it provides actual-time insights into how an application behaves, assisting you comprehend what’s taking place under the hood without needing to pause execution or step through the code line by line.
A good logging strategy starts off with being aware of what to log and at what stage. Frequent logging amounts contain DEBUG, Information, WARN, Mistake, and Deadly. Use DEBUG for thorough diagnostic details in the course of improvement, Information for common events (like successful get started-ups), Alert for prospective problems that don’t crack the appliance, ERROR for actual complications, and Deadly once the system can’t go on.
Avoid flooding your logs with too much or irrelevant knowledge. A lot of logging can obscure important messages and decelerate your program. Concentrate on vital functions, state variations, input/output values, and critical final decision points in the code.
Format your log messages Evidently and persistently. Consist of context, which include timestamps, request IDs, and performance names, so it’s simpler to trace issues in dispersed methods or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even easier to parse and filter logs programmatically.
Through debugging, logs Allow you to observe how variables evolve, what circumstances are fulfilled, and what branches of logic are executed—all with out halting This system. They’re especially worthwhile in production environments the place stepping through code isn’t attainable.
Additionally, use logging frameworks and equipment (like Log4j, Winston, or Python’s logging module) that aid log rotation, filtering, and integration with monitoring dashboards.
Eventually, intelligent logging is about stability and clarity. By using a well-imagined-out logging tactic, you are able to decrease the time it's going to take to spot concerns, get further visibility into your applications, and Enhance the Over-all maintainability and trustworthiness of one's code.
Assume Similar to a Detective
Debugging is not just a specialized process—it is a method of investigation. To successfully recognize and correct bugs, builders must method the process just like a detective fixing a thriller. This way of thinking allows break down complicated concerns into manageable areas and observe clues logically to uncover the foundation induce.
Start by gathering proof. Think about the symptoms of the issue: error messages, incorrect output, or overall performance concerns. Similar to a detective surveys a criminal offense scene, acquire as much pertinent facts as you may devoid of jumping to conclusions. Use logs, test cases, and user reports to piece together a transparent photograph of what’s occurring.
Upcoming, sort hypotheses. Question by yourself: What may be triggering this conduct? Have any alterations just lately been created towards the codebase? Has this problem happened in advance of underneath related situations? The objective is to slender down options and discover prospective culprits.
Then, check your theories systematically. Try to recreate the condition in the controlled environment. If you suspect a certain perform or component, isolate it and validate if The problem persists. Like a detective conducting interviews, question your code issues and Allow the results click here direct you closer to the reality.
Spend shut focus to small facts. Bugs usually disguise while in the least predicted places—similar to a missing semicolon, an off-by-just one error, or maybe a race situation. Be complete and individual, resisting the urge to patch The difficulty without having absolutely knowing it. Non permanent fixes could disguise the real dilemma, just for it to resurface later.
And lastly, keep notes on Whatever you tried using and realized. Equally as detectives log their investigations, documenting your debugging process can preserve time for upcoming problems and support others recognize your reasoning.
By wondering like a detective, developers can sharpen their analytical capabilities, solution issues methodically, and turn into more practical at uncovering concealed problems in sophisticated devices.
Generate Tests
Creating exams is among the simplest tips on how to improve your debugging techniques and In general development efficiency. Exams not merely support capture bugs early and also function a security Web that offers you assurance when earning changes to your codebase. A nicely-tested application is easier to debug because it enables you to pinpoint precisely exactly where and when an issue happens.
Start with unit checks, which deal with unique capabilities or modules. These smaller, isolated assessments can speedily reveal no matter whether a particular piece of logic is working as expected. Any time a take a look at fails, you promptly know the place to seem, substantially decreasing the time used debugging. Device exams are Particularly useful for catching regression bugs—challenges that reappear immediately after Earlier getting fixed.
Future, combine integration exams and end-to-conclusion exams into your workflow. These assist make sure several areas of your application get the job done collectively smoothly. They’re specially beneficial for catching bugs that occur in elaborate programs with multiple parts or providers interacting. If something breaks, your checks can let you know which Element of the pipeline failed and under what problems.
Writing assessments also forces you to Assume critically about your code. To check a function adequately, you'll need to be familiar with its inputs, anticipated outputs, and edge scenarios. This degree of knowledge Normally sales opportunities to better code framework and much less bugs.
When debugging an issue, producing a failing test that reproduces the bug could be a robust first step. After the take a look at fails regularly, it is possible to deal with fixing the bug and look at your exam pass when The problem is solved. This solution ensures that precisely the same bug doesn’t return Down the road.
In short, creating assessments turns debugging from the disheartening guessing sport into a structured and predictable approach—encouraging you catch far more bugs, a lot quicker and even more reliably.
Just take Breaks
When debugging a difficult difficulty, it’s easy to become immersed in the issue—watching your display screen for hrs, hoping Alternative just after solution. But Probably the most underrated debugging resources is just stepping away. Using breaks aids you reset your brain, minimize stress, and sometimes see The problem from a new viewpoint.
When you're as well close to the code for too long, cognitive exhaustion sets in. You might start overlooking noticeable faults or misreading code that you choose to wrote just several hours previously. In this particular condition, your brain becomes less economical at trouble-fixing. A short walk, a espresso split, and even switching to a special task for ten–quarter-hour can refresh your target. Numerous developers report getting the basis of an issue after they've taken time to disconnect, permitting their subconscious operate inside the background.
Breaks also enable avert burnout, Specifically throughout for a longer period debugging periods. Sitting before a display, mentally trapped, is not merely unproductive but also draining. Stepping absent permits you to return with renewed energy in addition to a clearer frame of mind. You might quickly recognize a lacking semicolon, a logic flaw, or possibly a misplaced variable that eluded you before.
When you’re stuck, a fantastic rule of thumb should be to set a timer—debug actively for forty five–60 minutes, then take a five–10 moment break. Use that time to maneuver about, extend, or do some thing unrelated to code. It could really feel counterintuitive, In particular under restricted deadlines, but it truly causes more quickly and more practical debugging In the end.
Briefly, having breaks just isn't an indication of weak spot—it’s a smart approach. It presents your brain Room to breathe, increases your perspective, and will help you steer clear of the tunnel vision That usually blocks your development. Debugging is usually a mental puzzle, and rest is a component of resolving it.
Master From Each and every Bug
Just about every bug you come upon is more than just A brief setback—It can be a possibility to grow like a developer. No matter if it’s a syntax mistake, a logic flaw, or a deep architectural problem, each one can educate you anything precious for those who make an effort to reflect and evaluate what went Erroneous.
Start by asking yourself a couple of crucial queries after the bug is solved: What induced it? Why did it go unnoticed? Could it are caught previously with superior techniques like device screening, code testimonials, or logging? The solutions typically reveal blind spots inside your workflow or comprehending and enable you to Construct stronger coding routines moving ahead.
Documenting bugs will also be a wonderful practice. Hold a developer journal or keep a log where you Be aware down bugs you’ve encountered, the way you solved them, and Anything you figured out. After some time, you’ll start to see patterns—recurring issues or common issues—you could proactively prevent.
In crew environments, sharing Everything you've learned from the bug using your peers is usually In particular strong. Regardless of whether it’s through a Slack concept, a short generate-up, or a quick understanding-sharing session, encouraging Other folks avoid the exact situation boosts group performance and cultivates a more powerful learning lifestyle.
Much more importantly, viewing bugs as classes shifts your state of mind from irritation to curiosity. As an alternative to dreading bugs, you’ll begin appreciating them as necessary elements of your enhancement journey. In any case, some of the ideal builders will not be those who compose fantastic code, but people who consistently discover from their faults.
In the end, Every single bug you fix adds a different layer for your ability established. So subsequent time you squash a bug, take a minute to reflect—you’ll arrive absent a smarter, extra capable developer on account of it.
Summary
Improving your debugging expertise usually takes time, practice, and persistence — although the payoff is large. It tends to make you a more successful, self-assured, and capable developer. The following time you happen to be knee-deep in a very mysterious bug, remember: debugging isn’t a chore — it’s an opportunity to become greater at Anything you do.